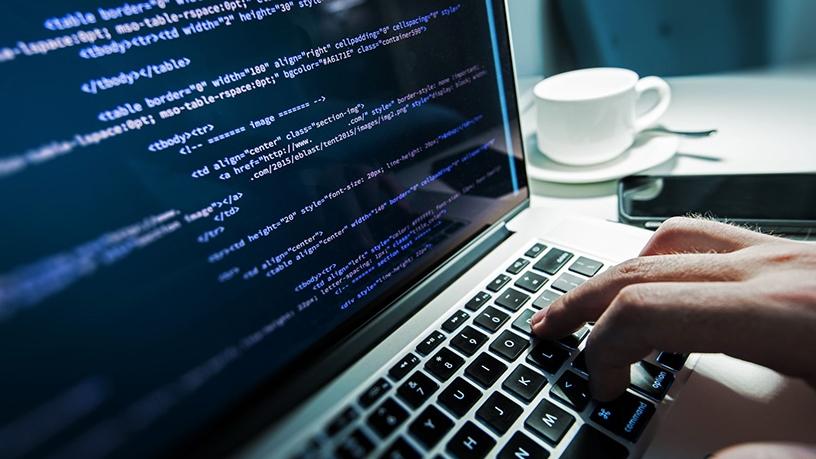
What is JSON And How To Using It In C#
Merhabalar.
Geçen hafta C# Corner adresinde yayınlanmış olan İngilizce makalemin orijinal kopyasını kendi web adresimde de paylaşmaya karar verdim. 11.04.2016 10:47 itibari ile 2.9k okunmasıyla beraber, aldığım geri bildirimler ve gelen mesajlar ile “Bir sonraki makale ne olsun?” sorusuna da cevap arıyorum. Herkese iyi kodlamalar.
Working with JSON in C#
(What is JSON And How to Using It In C#)
This article will cover the following:
- What is JSON
- How to create JSON string in C#
- How to read JSON string in C#
What is JSON
JSON (JavaScript Object Notation) is standard designed for human-readable data interchange. I think the thing of ‘human-readable’ for developers. JSON works with a tree structure and it looks like a XML. It’s shorter and It’s very easy for using. If you have already experience with XML, you will certainly learn easily.
JSON vs. XML
Let’s examine this expression { “name”: “Stephen Cousins” }
- If you’re using JSON, you must put your expression in “{ }”
- “name” is the key.
- “Stephen Cousins” is a value of “name” key
JSON has the logic like { “key”: “value” } statement.
And also JSON has the array.
Let’s take another expression and examine that:
"students": [ { "name": "Stephen Cousins" }, { "name": "Austin A. Newton" }, { "name": "Adam Wilhite" }, { "name": "Enis Kurtay YILMAZ" } ]
- “students” is the key.
- “[“ and “]” square brackets are array statement. It means there is the array between “[“ and “]” in brackets.
- All of statements in square brackets are value of “students” key.
- As you see there are four arrays in square brackets and it represents four student names.
How to create JSON string in C#
Please create your new console project from Visual Studio
File -> New-> Project->Console Application (.NET Framework 3.5)
If you want to create or read a JSON string, you need a JSON Serialize or Deserialize.
So, please open your Solution Explorer in Visual Studio, right click on References, click “Manage NuGet Packages”
Please search “Newtonsoft.JSON” on Nuget Package Manager and install it.
Please add “using Newtonsoft.Json;” statement. If you forget to add this statement, you can’t serialize any JSON strings.
Example JSON:
{ "universities": { "university": "South Carolina State University", "students": [ { "name": "Stephen Cousins" }, { "name": "Austin A. Newton" }, { "name": "Adam Wilhite" }, { "name": "Enis Kurtay YILMAZ"} ] } }
Now, you need to create class for example JSON. If you want to create easily, you can use that web site -> www.jsonutils.com
Creating JSON string in C#:
using System; using System.Collections.Generic; using Newtonsoft.Json; namespace EKY.CSharpCornerJSONArticle { public class Student { public string name { get; set; } } public class Universities { public string university { get; set; } public IList<Student> students { get; set; } } public class ClassUniversities { public Universities universities { get; set; } } class Program { static void Main(string[] args) { ClassUniversities university1 = new ClassUniversities(); university1.universities = new Universities(); university1.universities.university = "South Carolina State University"; List<Student> listStudent = new List<Student>(); Student student1 = new Student { name = "Stephen Cousins"}; Student student2 = new Student { name = "Austin A. Newton" }; Student student3 = new Student { name = "Adam Wilhite" }; Student student4 = new Student { name = "Enis Kurtay YILMAZ" }; listStudent.Add(student1); listStudent.Add(student2); listStudent.Add(student3); listStudent.Add(student4); university1.universities.students = listStudent; string json = JsonConvert.SerializeObject(university1); Console.WriteLine(json); Console.ReadLine(); } } }
Result.
How to read JSON string in C#
I think this part will be very easy for you. Just you need an example JSON string and JSON class of your example. You can use www.jsonutils.com again, if you want to create a class for your example JSON.
I will JSON strings from my website -> https://www.eniskurtayyilmaz.com/api/get_posts/
using System; using System.Collections.Generic; using Newtonsoft.Json; using System.Net; using System.IO; namespace EKY.CSharpCornerJSONArticle { public class Category { public int id { get; set; } public string slug { get; set; } public string title { get; set; } public string description { get; set; } public int parent { get; set; } public int post_count { get; set; } } public class Tag { public int id { get; set; } public string slug { get; set; } public string title { get; set; } public string description { get; set; } public int post_count { get; set; } } public class Author { public int id { get; set; } public string slug { get; set; } public string name { get; set; } public string first_name { get; set; } public string last_name { get; set; } public string nickname { get; set; } public string url { get; set; } public string description { get; set; } } public class CustomFields { } public class Post { public int id { get; set; } public string type { get; set; } public string slug { get; set; } public string url { get; set; } public string status { get; set; } public string title { get; set; } public string title_plain { get; set; } public string content { get; set; } public string excerpt { get; set; } public string date { get; set; } public string modified { get; set; } public IList<Category> categories { get; set; } public IList<Tag> tags { get; set; } public Author author { get; set; } public IList<object> comments { get; set; } public IList<object> attachments { get; set; } public int comment_count { get; set; } public string comment_status { get; set; } public CustomFields custom_fields { get; set; } } public class Query { public bool ignore_sticky_posts { get; set; } } public class ClassWebsiteposts { public string status { get; set; } public int count { get; set; } public int count_total { get; set; } public int pages { get; set; } public IList<Post> posts { get; set; } public Query query { get; set; } } class Program { static void Main(string[] args) { string url = "https://www.eniskurtayyilmaz.com/api/get_posts/"; HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest; string jsonValue = ""; using (HttpWebResponse response = request.GetResponse() as HttpWebResponse) { StreamReader reader = new StreamReader(response.GetResponseStream()); jsonValue = reader.ReadToEnd(); } ClassWebsiteposts websitePosts = JsonConvert.DeserializeObject<ClassWebsiteposts>(jsonValue); } } }
Result.
All of source code for downloading http://download.eniskurtayyilmaz.com/csharpcornerarticle.rar